Web3data.js—The new web3.js & ether.js drop-in replacement→
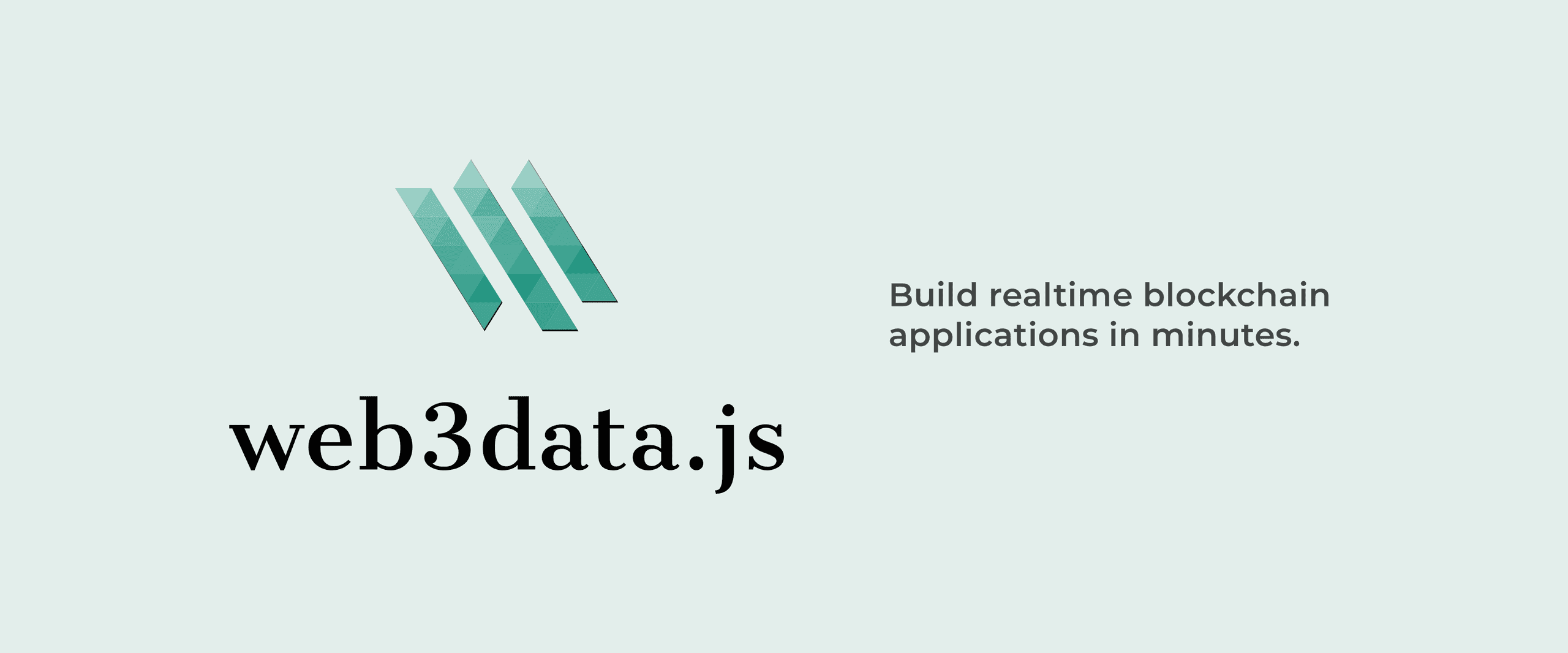
TLDR;
As easy as changing this:
web3.eth.getBlockNumber([callback])
to this
await web3data.eth.getBlockNumber()
Get the best blockchain data without the need to rewrite large amounts of code.
If you’d like to follow along and try out the code samples, you can quickly grab an API key and paste it in the code where it says YOUR_API_KEY
.
We want to make blockchain development as pain free as possible. That’s why we’ve worked hard to build helpful API’s and tools.
Migration
When migrating over from Web3.js or Ethers.js, method names and parameters will remain the same. Refer to the description below depending on which library you’re moving away from.
Coming from Web3.js?
Let’s say we want to get a transaction from a block with web3.js
:
let transaction = web3.eth.getTransactionFromBlock( 8000000, // block number 2 // transaction index)console.log(transaction)
With web3data.js
it will look like this:
let transaction = web3data.eth.getTransactionFromBlock( 8000000, // block number 2 // transaction index)console.log(transaction)
Coming from Ethers.js?
This is a similar process as above. We’re going to get a transaction by hash.
let transaction = await provider.getTransaction('0xc0s2d1bea...')console.log(transaction)
With web3data.js
it will look like this:
let transaction = await web3data.eth.getTransaction('0xc0s2d1bea...')console.log(transaction)
About the same right? However, the main difference is in the breadth of transaction data returned. web3data.js
will return additional metrics such as:
- The status of the transaction —
success
orfail
- Whether it’s confirmed or not along with the number of confirmations
- The fee associated with the transaction
Furthermore, it’s possible to include the price data with the transaction giving you the price in USD (or other currency) of the value that was transfered. That’s powerful!
{ "blockNumber": "7984429", "confirmations": "463134", "contractAddress": "null", "cumulativeGasUsed": "21000", "fee": "861000000000000", "from": { "address": "0xdb725da5de68db403de513936050da2a4a743185" }, "gasLimit": "21000", "gasPrice": "41000000000", "gasUsed": "21000", "hash": "0xd0a5a0912fdf87993b3cebd696f1ee667a8fbbe8fc890a22dcbdf114f36de4cf", "index": 0, "input": "0x", "logsBloom": "0x0000000000...", "nonce": "2337", "publicKey": "", "r": "0xbe443c97cdb4f923d9557bd84b538da10ad171b2748c5670f165e9beba8b0bdd", "raw": "", "root": "", "s": "0x656cae3d42798a3460917cfbab6a3cf28b1776373ad2107560dc9eb77bb4ac5e", "status": "0x1", "timestamp": "2019-06-18T20:23:14.000Z", "to": [ { "address": "0x4929d4a08be7d47081b56a7cd020ba34a629895b" } ], "v": "27", "value": "4000000000000000", "statusResult": { "code": "0x1", "confirmed": true, "success": true, "name": "successful" }, "price": { "value": { "currency": "usd", "quote": "265.179022118", "total": "1.06071608847200000000000000000" } }}
Why Web3data.js?
We wanted to ease the burden on developers interested in switching to Amberdata’s powerful API and lower the barrier to entry.
In most cases, it’s as easy as changing a variable name.
web3data.js
replaces existing web3.js
/ethers.js
methods but comes with Amberdata’s feature rich API. We’ll cover some of the reasons why you might want to opt in to the these additional features that we offer.
Websockets
Responding to real-time blockchain data can be an integral part of any dApp. Traditionally, this has been somewhat of a challenge for developers to set up. The experience of working with real time blockchain data should be no different than conventional websocket implementations.
That’s why created a rich yet simple websocket API inside our intuitive Javascript wrapper.
For instance, if you want to get a notification for the next new block:
Change .once()
to .on()
and it will get every new block.
Quick and easy.
Account balance + context
If you just want the address balance then web3data.getBalance()
works great. However, what these other libraries are not able to offer is context.
Using Web3data.js you’ll be able to get much more than an addresses balance. For instance:
This will return data like so:
{ “balance”: “69498477186533922505”, “balanceIn”: “2.7938614849190848921864e+22”, “balanceOut”: “2.7869116372004314999359e+22”, “addressType”: “contract”, “contractTypes”: [ “ERC721” ], “decimals”: “0”, “name”: “CryptoKitties”, “numHolders”: “73880”, “numTokens”: “1512179”, “numTransfers”: “1557375”, “symbol”: “CK”, “totalSupply”: “1512179.0000000000000000”, “totalValueUSD”: null, “unitValueUSD”: null}
As you can see, we get a whole lot more than just a balance. We can see how much was transferred in and out, the type of address, in this case it’s the Crypto Kitties contract, and additional information about the contract.
We can also see the balance in USD by specifying includePrice:true
in the filters:
Then we’ll having the pricing data attached with the request:
{ "price": { "balance": { "currency": "usd", "quote": "169.421541883", "total": "101179.2152367673770563829777385" } }}
This shows us that the balance of the account is $101,179.22. At the rate of 1 Eth = ~$169.42. The combination of market data + blockchain data is game changing.
Validating Transactions
Normally we can get any transaction by calling, web3data.eth.getTransaction
and that will return the transaction hash & value, block information, addresses to and from, etc.
However, we have no way of validating that transaction as we aren’t given the necessary data components. If we want to get the full transaction context along with ability to validate said transaction we can use web3data.transaction.getTransaction
:
This will return loads more data than before! Now we can use these data points to prove the validity of the transaction.
Where’s this data coming from?
Currently, we have limited options when it comes to building modern and fast dApps — By running your own node or using a node provider i.e. Node Smith. The blockchain is great for security and data integrity but is nowhere near as efficient as present database solutions such as SQL or document based data stores nor does it offer the advanced querying capabilities.
What we have done at Amberdata is create one of the most extensive blockchain data platforms offering additional metrics far beyond the capacity of the traditional JSON RPC interface.
Here’s a list of currently supported web3.js
/ethers.js
methods:
Stay Tuned!
We’ll be releasing more tutorials on how you can integrate web3data.js
into your dApp!