Same code, no node. Web3data.js is the easiest blockchain library, available now!
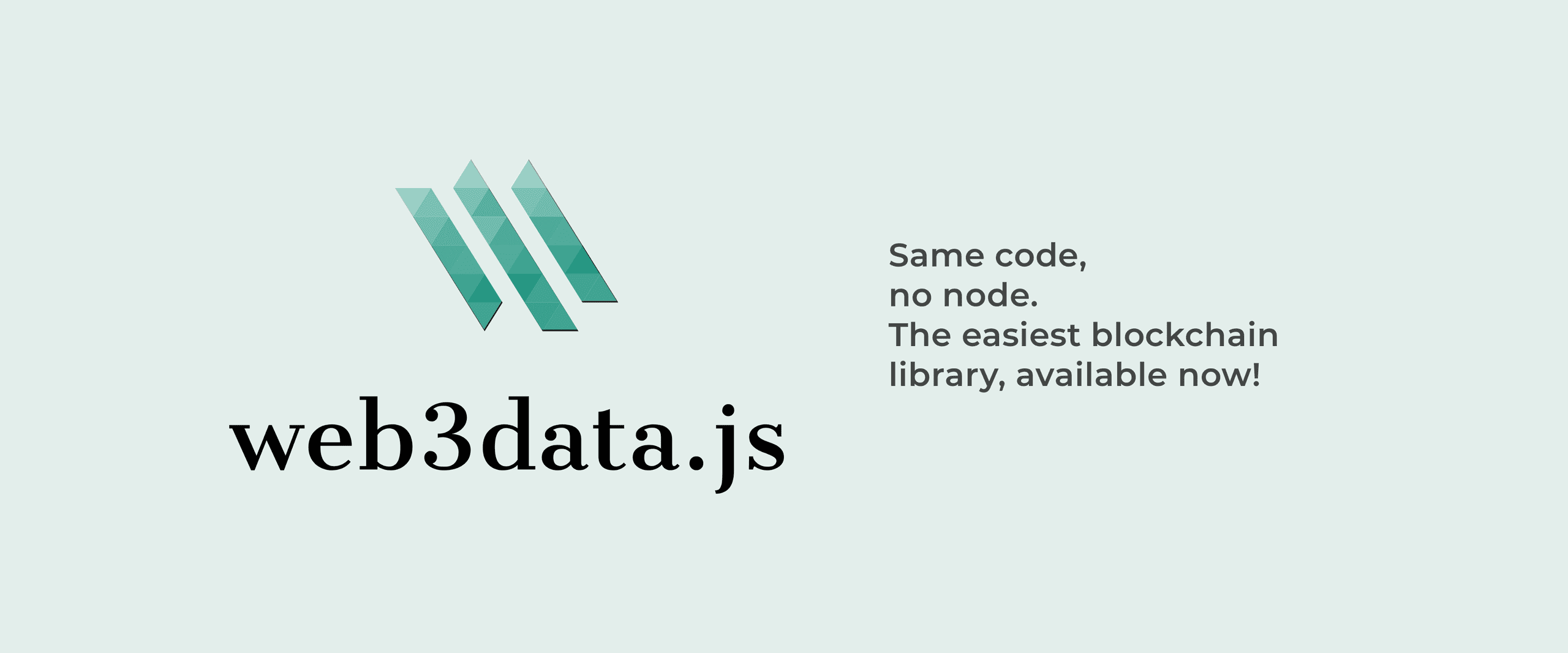
Web3data.js is RPC compliant so you can have the benefits of Amberdata’s advanced & comprehensive API with the convenience & compatibility of RPC.
TLDR;
We’re excited to announce that Web3data.js now has JSON RPC support!
web3data.<blockchain>.rpc(<method_name> [, params])
Web3data.js just got a whole lot better.
If you’d like to follow along and try out the code samples, you can quickly grab an API key and paste it in the code where it says YOUR_API_KEY
.
It’s now possible to use JSON RPC methods, as if you’re interacting directly with a blockchain node. We did this to bring the traditional and familiar functionality that comes with pre-existing JSON RPC methods. This should make it much easier to incorporate into existing codebases that are already built using these methods.
Therefore, integrating Web3data.js into your existing projects (or if you’re just starting out) doesn’t require rewriting numerous lines of code but rather allows us to begin focusing on using the more powerful features that come with the library.
Usage
Here’s the basic syntax:
web3data.<blockchain>.rpc(<method_name>[, params])
blockchain
— Namespacing is used to specify the blockchain. Omitting this value will default to Ethereum Mainnet.method_name
— The RPC method which to call e.g.eth_blockNumber
params
— These are the parameters to the RPC method. It can either be an array or a single value. Web3data.js will make sure it’s in the correct format before it’s shipped off.
Note: Upon instantiation of Web3Data it is possible to specify a blockchainId
. When using the namespaced methods, the specified blockchainId
will be overridden. For instance:
const w3d = new Web3Data(API_KEY, {blockchainId: 'ETH_RINKEBY_ID'})w3d.rpc('eth_blockNumber') // Ethereum Rinkeby's block numberw3d.eth.rpc('eth_blockNumber') // Ethereum Mainnet's block number
Here’s an example of getting the transaction count of an address using eth_getTransactionCount:
await web3data.eth.rpc("eth_getTransactionCount", ["0x...", "latest"]).then(console.log)// RPC response{ "jsonrpc": "2.0", "id": 1, "result": "0x1a" // 26}
You can view a list of supported methods in our docs. Or by calling:
web3data.eth.rpc('amb_supportedMethods')
Estimating Gas
Before deploying that 500 line contract that will power you’re dApp, it’s useful to know how much gas it will take to execute the transaction. Using eth_estimateGas we’re able to get an estimate of how much gas it will cost.
web3data.eth.rpc('eth_estimateGas', [{ "from":"0xb60e8dd61c5d32be8058bb8eb970870f07233155", "to":"0xd46e8dd67c5d32be8058bb8eb970870f07244567", "gas":"0x76c0", "gasPrice":"0x9184e72a000", "value":"0x9184e72a","data":"0xd46e8dd67c5d32be8d46e8dd67c5d32be8058bb8eb970870f072445675058bb8eb970870f072445675"}])
Sending Raw Transactions
We can use Web3data.js in combination with ethereumjs-tx & ethereumjs-util to construct, sign, and send a transaction. Below you’ll find a code example with verbose commenting. If you pull the gist, and fill in necessary data you can run this example. As always, please exercise caution whenever you’re dealing with private. In addition, make sure you are an the correct network and sending to/from the right address when executing the transaction, so as not inadvertently “burn” your funds.
JSON RPC or API?
JSON RPC is the standard way to retrieve data from the blockchain and you can get a lot of raw data this way. However, there are many cases where opting for the API, via Web3data.js built in methods, can yield better more usable data and increased context. The API can also provide data that is aggregated and would otherwise be hard or time consuming to obtain.
Web3data.js has methods that provide these aggregations with ease of use.
Getting an account balance
Using eth_getBalance
we can obtain the balance of any account like so:
w3d.eth.rpc('eth_getBalance', ['0xc94770007dda54cF92009BFF0dE90c06F603a09f', 'latest'])//// RPC Response{ "jsonrpc": "2.0", "id": 1, "result": "0x16bcc41e90000"}
Great! We got the account’s balance but what if we wanted to also know the balance in USD? We could take that value, convert it to decimal, then from wei to Eth and finally multiply it by the current exchange rate.
Or what if we wanted to get historical time series balances for that same address? We would most likely need to make a great deal of RPC requests
There’s actually an easier way:
web3data.address.getBalance( "0xc94770007dda54cF92009BFF0dE90c06F603a09f", {includePrice: true})
We can use Web3data.js’s getBalance
method to return not only the balance of the account in wei but also the balance in USD. You can do this by specifying includePrice: true
.
And we get the historical account balance using the same method by specifying a startDate
and/or endDate
. Web3data.js automagically knows if you want historical or current account balance based on the parameters.
w3d.address.getBalance("0xc94770007dda54cF92009BFF0dE90c06F603a09f",{startDate: 1556184430})
Here’s a full example you could try:
Getting a contract’s code
Using eth_getCode
we can get the byte code of a contract like so:
web3data.eth.rpc('eth_getCode', ['0x06012c8cf97bead5deae237070f9587f8e7a266d', 'latest'])
This will return the byte code for Cryptokittes!😸 But now let’s say we would like the decompiled byte code? Or even the source code? How about the contract’s ABI?
We can actually get all of this information using a single method:
web3data.contract.getDetails('0x06012c8cf97bead5deae237070f9587f8e7a266d'){ "abi": [{...}], "bytecode": "...", "contractName": "CryptoKitties", "source": "pragma solidity ^0.4.11;\n\n\n..."}
Boom! Almost everything we would need to know about a contract in a single method call. Now we could feed that into our dApp and start calling methods on the contract. You can view more details about the endpoint response in the docs.
Conclusion
That was a brief overview of some of the many ways you can utilize the power of raw JSON RPC methods with Web3data.js. Let us know how you’re using Web3data.js and or if we can help in anyway!
We love hearing from our users so don’t hesitate to reach out. :)
Stay Tuned!
We’ll be releasing more tutorials on how you can integrate web3data.js
into your dApp!